The power supplies module¶
Abstraction module for power supplies (PS). The ps module (cmd_ps) allows the transparent use of a pool of an undefined number of PS regardless of maker, model or host-device link technology. Its function is to redirect the requests and orders made to it, to a module that knows how to interface with that particular PS. That module can in turn use one of the available lower-level interfacing modules (GPIB, TCP, serial).
As of this writing, modules for the following PS are implemented:
On the following, the ps module and the way to implement new PS modules is described. For information on how to interface with the bus modules, refer to their documentation.
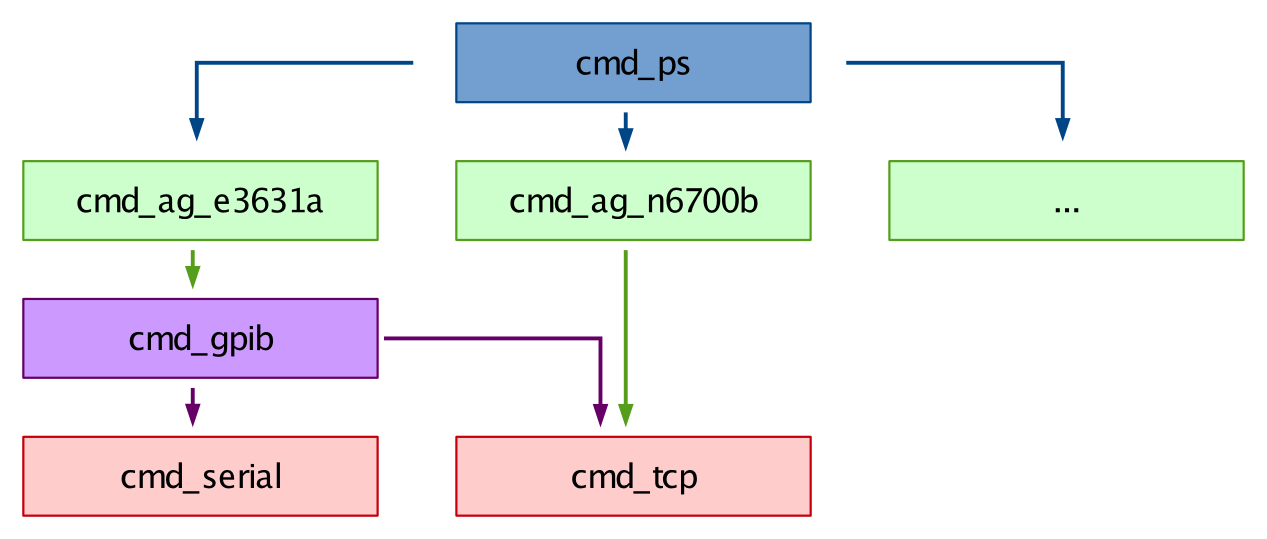
Figure 1: Example of interaction between cmd_ps, PS modules and pyrame bus modules (gpib, serial, tcp).
Pool and identification¶
Every PS in the pool is identified uniquely by an identifier passed to the function init_ps
. All the other functions require this id token as the first argument.
During init_ps, the initialization function for the particular model is called. Its name must be init_MODEL (e.g.: init_ag_e3631a
). The convention style for MODEL is a two-letter maker symbol plus the model, separated by an underscore (_).
Initialization¶
As pointed out previously, cmd_ps provides a function init_ps and requires functions init_MODEL from the modules with which it interfaces. The init_ps declaration is:
-
init_ps
(ps_id, conf_string)¶ Registers in the pool and initializes a new PS with ps_id. conf_string is the configuration string for the module to be initialized. On PS supporting different channels, the conf_string must include the parameter chan to indicate the channel that will be used. An identificator will be needed for each channel.
Functions¶
A series of functions in cmd_ps interface the corresponding functions on the PS modules. In most cases, a call to the function of the same name is performed (replacing _ps by _MODEL). All PS models must include, at least, an implementation of the following functions:
- init_MODEL (model_id,*conf_string*)
- deinit_MODEL (model_id)
- config_MODEL (model_id)
- inval_MODEL (model_id)
Optionally, these functions can also be implemented:
- set_voltage_MODEL (model_id, voltage [, slew_rate])
- set_current_MODEL (model_id, current)
- power_on_MODEL (model_id)
- power_off_MODEL (model_id)
- get_voltage_MODEL (model_id)
- get_current_MODEL (model_id)
- set_voltage_limit_MODEL (model_id, voltage_limit)
- set_current_limit_MODEL (model_id, current_limit)
- set_rise_delay_MODEL (model_id, rise_delay)
- get_error_queue_MODEL (model_id)
In all cases:
- Magnitudes (i.e.: voltage, current, slew_rate, etc.), either sent or returned, must be expressed in base units of the International System of Units (V, A, V/s, etc.).
The functionality provided by each of the functions is primarily the following:
set_voltage_MODEL (model_id, voltage [, slew_rate]])
Set voltage on PS at the specified slew rate. A previous call to set_current_limit is necessary.set_current_MODEL (model_id, current)
Set current on PS. A previous call to set_voltage_limit is necessary.power_on_MODEL (model_id)
Power on PS.power_off_MODEL (model_id)
Power off PS.get_voltage_MODEL (model_id)
Get voltage from PS. Preferentially measured, not setpoint reading.get_current_MODEL (model_id)
Get current from PS. Preferentially measured, not setpoint reading.set_voltage_limit_MODEL (model_id, voltage_limit)
Set voltage limit. When available, use a Over Voltage Protection setting.set_current_limit_MODEL (model_id, current_limit)
Set current limit. When available, use a Over Current Protection setting.set_rise_delay_MODEL (model_id, rise_delay)
Set delay between receiving power-on command and actually engaging.get_error_queue_MODEL (model_id)
Get queue of errors.
Note
As hinted out by the optional arguments on the list of functions, the arguments passed through to the model function will depend on the capabilities of that particular PS model. cmd_ps uses the API exchange mechanism to determine them.
API¶
-
cmd_ps.
init_ps
(ps_id, conf_string)[source]¶ Registers in the pool and initializes a new PS. conf_string is the configuration string for the module to be initialized.
-
cmd_ps.
set_voltage_ps
(ps_id, voltage, slew_rate='undef')[source]¶ Set voltage in Volts. Optional slew_rate argument in V/s for PS modules that support it.
-
cmd_ps.
set_digital_on_ps
(ps_id, channel='undef', active_level='undef')[source]¶ Turn on digital output on PS