See pyLikelihood:
Also see:
|
Interactively Explore
pyLikelihood Functions
You can perform a pyLikelihood analysis from the python prompt, which is useful in testing a new command and its parameters before incorporating it into a script file. Or, you may want to perform some functions interactively after performing an analysis (e.g., you may want to unset and change a fixed parameter).
For the purposes of continuity, we'll perform the same UnbinnedAnalysis that we performed in the pyLikelihood: UnbinnedAnalysis section of this tutorial, but this time from the python prompt.
Prerequisites
Remember: We ran the "my_unbinned_analysis" script from our mypyLike work directory and used the following files:
- FT1.fits file - 3C454_back-filtered.fits
- FT2.fits file - pyLike-ft2-30s.fits
- expMap.fits - 3C454-expMap.fits
- expCube.fits - 3C454-expCube.fits
- IRFs - P6_V3_DIFFUSE
Create an UnbinnedObs object
- Log in to SLAC Central Linux and change to your work directory (e.g., mypyLike).
Note: If you are running on a Windows machine, use X-Win32 as your X server.
- Set up your SCons environment; see Setup SLAC Central Linux.
- At the prompt, enter: python.
- From the python prompt, import the data and analysis classes:
>>> from UnbinnedAnalysis import *
- Create an UnbinnedObs object; for example, the UnbinnedObs constructor is:
my_obs = UnbinnedObs('FT1.fits', 'FT2.fits', 'expMap.fits', 'expCube.fits', 'P6_V3_DIFFUSE'); so, from the python prompt, enter:
>>>likeObs = UnbinnedObs('3C454_back-filtered.fits', 'pyLike-ft2-30s.fits', '3C454-expMap.fits', '3C454-expCube.fits', 'P6_V3_DIFFUSE')
Note: FT1 event .fits file(s) (i.e., the 3C454_back-filtered.fits file shown above); can be entered as:
- A single .fits file name (e.g., 3C454_back-filtered.fits)
- An ascii file containing the names of the event files; for example:
glitch [43] [cillis]: cat eventFiles
eg_dif_filtered.fits
galdif_filtered.fits
ptsrcs_filtered.fits
glitch [44] [cillis]:
Note: You can also select an Event-List text file when requesting data from the Astro Server. (See Data Acces Help: Astro Server.)
- A tuple or list of file names; for example, the following are equivalent to those in the ascii file shown above:
my_obs = UnbinnedObs(('eg_dif_filtered.fits', 'galdif_filtered.fits',
'ptsrcs_filtered.fits'),
'FT2.fits', expMap='expMap.fits',
expCube ='expCube.fits', irfs='P6_V3_DIFFUSE')
- You could also have entered in or use glob to generate the list; for example, the following are equivalent to the above:
>>> my_obs = UnbinnedObs(glob.glob('*filtered.fits'), 'FT2.fits', expMap='expMap.fits',
expCube='expCube.fits', irfs='P6_V3_DIFFUSE')
Also note: You can omit all of the arguments in the UnbinnedObs class constructor, in which case a small GUI dialog is launched that allows you to:
- Browse the file system
- Use wild cards for specifying groups of files,
- etc.
For example:
>>> my_obs = UnbinnedObs()
launches this dialog:
The buttons on the left will open file dialog boxes using the value shown in the text entry fields as a filter. You can include wild cards or comma-separated lists of files in the text entry field.
Setting the entries as follows will create an UnbinnedObs object equivalent to the previous examples:

|
Create an Instance of UnbinnedAnalysis
- From the python prompt, enter:
>>>like = UnbinnedAnalysis(likeObs, srcModel="3C454-srcModel.xml")
The srcModel.xml is the xml file containing the model definition for Likelihood; see Create a Source Model XML File (using Astro Data Viewer).
Note: If you omit the name of the srcModel.xml file, a small GUI is will be displayed that allows you to browse the file system; for example:
>>> analysis = UnbinnedAnalysis(my_obs)
launches:
|
View the UnbinneObs and UnbinnedAnalysis Classes
- To view the UnbinnedObs class, enter:
>>> print likeObs
Event file(s): 3C454_back-filtered.fits
Spacecraft file(s): pyLike-ft2-30s.fits
Exposure map: 3C454-expMap.fits
Exposure cube: 3C454-expCube.fits
IRFs: P6_V3_DIFFUSE
>>>
- To view the UnbinnedAnalysis class enter:
>>> print like
Event file(s): 3C454_back-filtered.fits
Spacecraft file(s): pyLike-ft2-30s.fits
Exposure map: 3C454-expMap.fits
Exposure cube: 3C454-expCube.fits
IRFs: P6_V3_DIFFUSE
Source model file: 3C454-srcModel.xml
Optimizer: Drmngb
>>>
Note that the Optimizer is Drmngb, but we want MINUIT.
- Enter:
>>>like.optimizer="MINUIT"
Then enter:
>>> print like
Event file(s): 3C454_back-filtered.fits
Spacecraft file(s): pyLike-ft2-30s.fits
Exposure map: 3C454-expMap.fits
Exposure cube: 3C454-expCube.fits
IRFs: P6_V3_DIFFUSE
Source model file: 3C454-srcModel.xml
Optimizer: MINUIT
>>>
- Enter:
>>>print like.fit(covar=True)
Note that the fit is: 4233.53736657
- Enter:
>>>like.plot()
Note: If you're running on a Windows machine, be sure that you are using XWin-32 as your X Server.
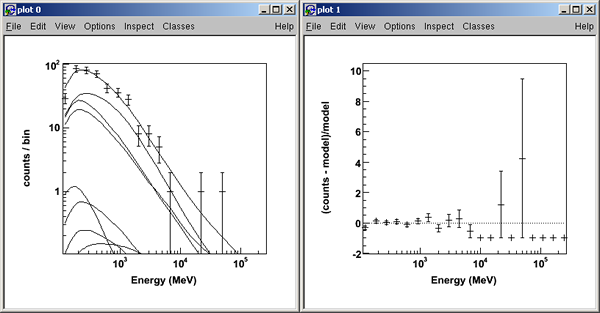
- Enter:
>>> like.model
4233.53736657 >>> print like.model EG_v02 Spectrum: FileFunction 0 Normalization: 6.499e-01 1.453e-02 1.000e-05 1.000e+03 ( 1.000e+00)EMS1499 Spectrum: PowerLaw2
1 Integral: 4.097e-01 1.459e+00 1.000e-05 1.000e+03 ( 1.000e-06)
2 Index: -3.355e+00 4.059e-01 -5.000e+00 -1.000e+00 ( 1.000e+00) 3 LowerLimit: 2.000e+01 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed 4 UpperLimit: 2.000e+05 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed EMS1505
Spectrum: PowerLaw2 5 Integral: 6.184e-01 6.095e-02 1.000e-05 1.000e+03 ( 1.000e-06) 6 Index: -2.207e+00 3.388e-02 -5.000e+00 -1.000e+00 ( 1.000e+00)
7 LowerLimit: 2.000e+01 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed
8 UpperLimit: 2.000e+05 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed EMS1517 Spectrum: PowerLaw2
9 Integral: 3.228e-01 2.105e+01 1.000e-05 1.000e+03 ( 1.000e-06) 10 Index: -4.360e+00 1.455e+01 -5.000e+00 -1.000e+00 ( 1.000e+00) 11 LowerLimit: 2.000e+01 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed 12 UpperLimit: 2.000e+05 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed EMS1524
Spectrum: PowerLaw2
13 Integral: 3.515e+01 4.167e-01 1.000e-05 1.000e+03 ( 1.000e-06) 14 Index: -2.444e+00 5.006e-03 -5.000e+00 -1.000e+00 ( 1.000e+00) 15 LowerLimit: 2.000e+01 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed 16 UpperLimit: 2.000e+05 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed EMS1525 Spectrum: PowerLaw2 17 Integral: 4.435e-02 5.063e-03 1.000e-05 1.000e+03 ( 1.000e-06) 18 Index: -1.405e+00 3.342e-02 -5.000e+00 -1.000e+00 ( 1.000e+00) 19 LowerLimit: 2.000e+01 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed 20 UpperLimit: 2.000e+05 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed EMS1536 Spectrum: PowerLaw2 21 Integral: 1.480e-01 2.304e-02 1.000e-05 1.000e+03 ( 1.000e-06) 22 Index: -1.955e+00 4.924e-02 -5.000e+00 -1.000e+00 ( 1.000e+00) 23 LowerLimit: 2.000e+01 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed 24 UpperLimit: 2.000e+05 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed EMS1538 Spectrum: PowerLaw2 25 Integral: 4.377e-01 1.371e+00 1.000e-05 1.000e+03 ( 1.000e-06) 26 Index: -4.678e+00 2.863e+00 -5.000e+00 -1.000e+00 ( 1.000e+00) 27 LowerLimit: 2.000e+01 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed 28 UpperLimit: 2.000e+05 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed EMS1562 Spectrum: PowerLaw2 29 Integral: 8.451e+00 1.016e+00 1.000e-05 1.000e+03 ( 1.000e-06) 30 Index: -3.446e+00 5.545e-02 -5.000e+00 -1.000e+00 ( 1.000e+00) 31 LowerLimit: 2.000e+01 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed 32 UpperLimit: 2.000e+05 0.000e+00 2.000e+01 2.000e+05 ( 1.000e+00) fixed GAL_v02 Spectrum: ConstantValue 33 Value: 1.315e+00 1.411e-02 0.000e+00 1.000e+01 ( 1.000e+00) >>>
|
Note: The columns are:
- parameter index
- parameter name
- parameter value
- error estimate (zero before fitting)
- lower bound
- upper bound
- parameter scaling (in parentheses)
- fixed (or not)
|
- You can change parameters using the index; for example (only), enter:
>>>like[33]=1.100
>>>like.fit(verbosity=0)
4233.5350369330818
Note that the fit has changed from 4233.53736657 in step 10, above.
- Enter:
>>>like.oplot()
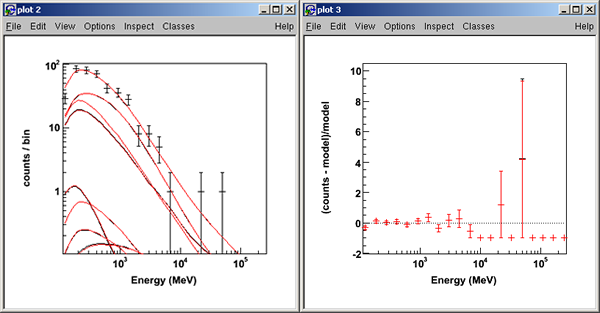
- Refer to pyLikelihood Attributes and Methods for additional functions that you can perform.
gt_apps
There are three key parts to gt_apps:
For an example of a script using gt_apps, see: gt_apps python Script Used in Binaries RSP Analysis.
For more examples of more scripts, see Richard Dubois' confluence page "Scripts used in Binaries RSP Analysis".
Please note: In future versions of this tutorial, it is our intent to include additional links to useful scripts that have been developed by subject matter experts.
Later, it may be useful to consolidate these scripts into a user-friendly catalog.
Last updated by: Chuck Patterson
04/01/2011 |
|
|